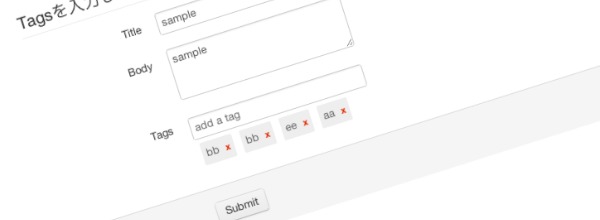
CakePHPでタグ機能を実装する際に便利そうなtag_timeというプラグインがあったので試してサンプルを作ってみました。
サンプルイメージ
作成するのはこんな画面です。
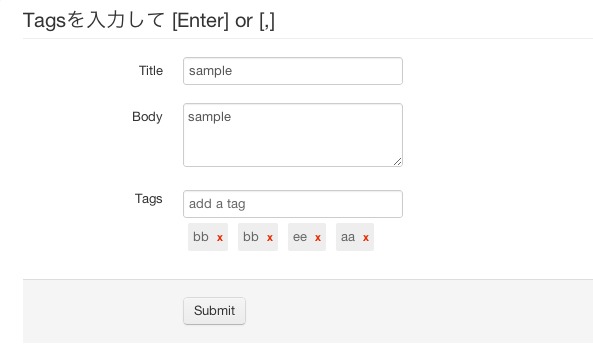
環境
CakePHP2.1.2
PHP5.3.8
TwitterBootstrap
※サンプルはTwitterBootstrapが前提になっています。
テーブル作成
こんな感じでタグを含むテーブル構成を作成します。
プラグイン配置
GitHubからプラグインをダウンロードし、プラグインフォルダに配置します。
https://github.com/voidet/tag_time/tree/2.0
bootstrapでプラグインを読み込みます。
Controller
コントローラでは何も難しいことはしません。
bakeした状態で問題ないと思います。
Model
モデルの$actsAsでプラグインを指定します。
忘れずにhasAndBelongsToManyも指定します。
View
Viewも特に変更してませんが、Tagの箇所だけ注意してください。
これで完成です。
簡単ですね。ほとんど自分ではタグに関するソースを書いてません。
表示のカスタマイズ等はcssで出来そうでした。
作成したサンプルはここ
タグの箇所を入力して確認してみてください。
http://sample.b-prep.com/posts
tag time